Fundamentals of Python Programming
In this article, we discussed the fundamentals of Python programming language which you need to know before writing simple python programs. This article describes how Python programs should work at most basic level and give details about operators, variables, identifiers, expressions and keywords upon which complex solution can be built.
Learning Objectives:
- Fundamentals of Python Programming Comments
- Fundamentals of Python Programming IDENTIFIERS
- Fundamentals of Python Programming KEYWORDS
- Fundamentals of Python Programming VARIABLES
- Fundamentals of Python Programming EXPRESSION AND STATEMENT
- Assignment Statement
- RECEIVING INPUT FROM KEYBOARD
1.1 Fundamentals of Python Programming Comments
As programs get bigger and complicated, they become more difficult to read. It is very difficult for us to look at a piece of code and figure out what is doing. For this reason, it is better to add text with programs in natural/simple language to explain what the program is doing.
In Python, a comment is just a piece of text that is intended only for human readers, and it is completely ignored by compilers and interpreters. A comment describes what is being done in a block of code. It does not affect the outcome of a code. Comments are used to increase the readability of code. They should be precise and clear.
Types of comments:
There are two types of comments in Python.
- Single line comment
- Multi-line comment
1. Single line comment:–
In Python, we use a # Hash token to start the comment. Here the symbol (#) is also known as octothorpe. When a # symbol is encountered anywhere in a program, everything after it will be ignored by the interpreter till the line ends. If # token is encountered inside quotes, then it is not considered as comment as shown below.
print (“#this is not a comment”) |
Output:
#this is not a comment |
2. Multi-Line comment:
When we extend commands in more than one line then each line should be prefixed by # token. Such comments are also known as block comments. Another efficient way to comment in a multiple lines is by using documentation strings to which we call DoctString in Python. Here we will make use of three single/double (“or””) quotes at the beginning and at the end of the comment.
Python comment examples:
This Python program demonstrates three types of comments – Single line comment, multi-line comment and the comment that is starting in the same line after the code which is known as inline comment.
1.2 Fundamentals of Python Programming IDENTIFIERS
A Python identifier is a name used to identify a variable, function, class, etc. an identify starts with a letter at A to Z or a to z or an underscore (_) followed by zero or more letters, underscores, and digits (0 to 9).
Python is a case sensitive programming language. Thus BOOK and book are two different identifiers in python.
Here are some naming conventions for python identifiers.
- Starting an identifier with two leading underscores indicates a strong private identifier.
- If the identifier also ends with two trailing underscores, the identifier is a language-defined special name.
- Starting an identifier with a single leading underscore indicate that the identifier is private.
- Class name starts with an uppercase letter. All other identifiers start with a lowercase letter.
Few examples of valid identifiers in Python are as follows:
- myClass
- var_1
- print_this_to_screen
- _abc
- Sub 1
1.3 Fundamentals of Python Programming KEYWORDS
In Python, keywords are the reserved words that have pre-defined meaning. Keywords are used by the Python interpreter to understand the program. Python does not allow us to use a keyword as a variable name, function name or any other identifier name. Keywords in python are case sensitive.
We can get the complete list of keywords using python interpreter help utility. There are 35 keywords in Python 3.7.3 release.
Table: List of keywords in Python
False | class | from | or |
None | continue | global | pass |
True | def | if | false |
Anddel | import | return | |
Aselif | in | try | |
Assertelse | is | while | |
Asyncexcept | lambda | with | |
Awaitfinally | nonlocal | yield | |
Breakfor | not |
Note: The above keywords may get altered in different versions of python. We can get the list of keywords in our current version by typing the following in the prompt.
1.4 Fundamentals of Python Programming VARIABLES
Variable is a memory space reserved in computer memory to store a value and that value can be changed further if needed. A variable can hold values of different data types. For example, a person name must be stored as a string. Where its ID must be stored as an integer. Based on the type of value, the interpreter allocates memory space.
1.4.1 Rules for naming Python variables:
- A variable name must start with a letter or the underscore(_). For example- var, Var_name, abc12, _XYZ etc.
- A variable name cannot start with a number.
- Variable names are case-sensitive (Book, Book and book are three different variables).
- A variable name can only contain alpha-numeric characters and underscores.
- Create a name that makes sense like age makes more sense than a.
- The reserved word (keywords) cannot be used for naming the variable.
1.4.2 How to create and use variables in Python:
- Python variable do not need explicit declaration to create a variable.
- The declaration happens automatically when value is assigned to a variable.
- The equal sign (=) is used to assign values to variables.
- The operand to the left of the = operator is the name of the variable and the operand to the right of the = operator is the value stored in the variable.
For example:
- Counter = 21 # Creating an integer variable and assigning integer value to it.
- Marks = 67.54 # Creating a floating variable and assigning floating value to it.
- Name = “Alice” # Creating a string variable and assigning a string value to it.
Note: As we know Python is completely object oriented every variable in Python is an object. In Python, we don’t actually assign values to the variables. Instead, Python gives the reference of the object (value) to the variable
1.4.3 Multiple Assignments:
- Python allows us to assign same value to multiple variables simultaneously. For example: Consider the following statement: x=y=z=3. This statement will assign values 3 to all the three variables.
- We can also assign different values to multiple variables simultaneously. For example: Consider the following statement: x,y,z =1.2,3, “ABC”. This statement will assign value 1.2 to x variable, value 3 to the y variable and a string ABC to the Z variable.
1.4.4 ID() Built-in Python function:
This function accepts a single parameter and returns the “identity” of an object. All objects in python have their own unique ID. This ID is assigned to the object when it is created. This identity is unique and constant for this object during the lifetime. Two objects with non-overlapping lifetimes may have the same ID(). If we compare this with C. then they are actually the memory address, here in python it is a unique ID. This function is generally used internally in python.
Syntax: ID(object)
Here parameter object can be any object, float, str, list, dict, tuple, class etc.
1.5 Fundamentals of Python Programming EXPRESSION AND STATEMENT
1.5.1 Expression:
Any sequence of operands and operators is called an expression or we can say it is a combination of values, variables, operators and called to functions. Expressions need to be evaluated and they always return a value. A value by itself is considered an expression, and so is a variable. Here are some examples of expressions.
- 2+2
- 1+2+3* (8**9) – sqrt (4.0)
- min (2,22)
- max (3,94)
- round (81.5)
- “Hello”
- “Learner”
- “Hello” + “Learner”
All of the above can be printed or assigned to a variable.
Evaluating and expressions is not quite the same thing as printing a value. The code below shows that when the Python interpreter displays the value of an expressions, it uses the same format we would use to enter a value. In case of strings, it includes the quotation marks. But if we use a print statement, Python displays the content of the string without the quotation marks.
Consider the following statements:

1.5.2 Statement:
A statement performs certain action (do something). It is an instruction that the Python interpreter can execute. A Python statement is made-up of one or more Python expressions.
Consider the following statements:
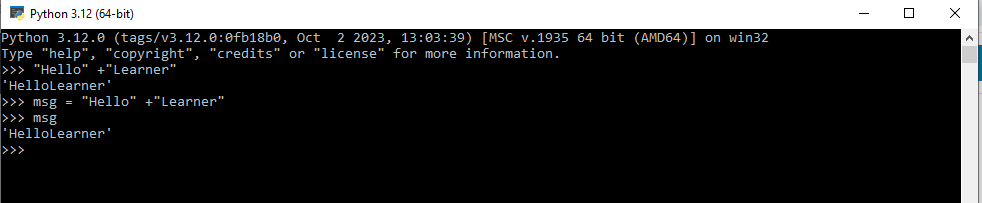
Here we are assigning the final evaluated expression value to another variable ‘msg’. This type of command where a value is assigned to a variable, is called as a Python statement.
Here are some examples of statements:
- if CONDITION :
- elif CONDITION :
- else :
- for VARIABLE in SEQUENCE :
- while CONDITION :
- try :
None of the above constructs can be assigned to a variable. They are syntactic elements that serve a purpose, but do not themselves have any intrinsic “value”. In other words, these constructs don’t “evaluate” to anything.
1.5.3 Difference between Expression and Statement:
Expression | Statement |
Represent something | Does something |
Python evaluates it | Python execute it |
Results in a value | Results in an action |
Example: 1. 2+3 | Example: 1. If x>7 |
2. “Hi” | 2. x=y+2 |
Note: Here the Thumb rule is – If we are able to print it, or assign it to variable, then it’s an expression. If we can’t, then it’s a statement.
1.6 Assignment Statement:
In assignment statement assigns a value to a variable by using an assignment operator (=).
For example:

This example makes three assignments. The first assigns the string “Hello Learner” to a new variable named msg. The second gives the integer 12 to age, and the third gives the floating point numbers 2.5 to radius.
The assignment operator (=) should not be confused with an equals sign used in mathematics. Assignment operators link a name, on the left hand side of the operator with a value on the right hand side. This is why we will get an error if we write.

One particularly important type of assignment statement looks like this: a=a+2. In mathematics this sentence is always false, since no number is equal to itself plus 2. However, in any programming language, here is what it means:
- Take the current value of a (Let a = 3).
- Add 2 to the current value i.e. 3 + 2 = 5.
- Put this evaluated value 5 back in memory location a, it will over-write the value that was there before.
1.7 RECEIVING INPUT FROM KEYBOARD
In Python, the built-in function input() is used to receive input from the keyboard. It waits for the input till your enter some value from keyboard followed by enter key and returns the entered value as string.
As we know this input() function returns entered value as a string. So to receive an integer/float value from user we need to convert value returned from input() function into integer/float with the help of built-in function int()/ float() respectively
1.8 Fundamentals of Python Programming OPERATOR
Operator is either a special symbol or keyword used to perform operation or variable or value. There are plenty of operators available in Python to perform different kind of operations. The value or variable on which operation is performed is called the operand.
Consider the expression:

Here 2and 3 are operands and + is the operator.
Python language supports a wide range of operators. They are as follows.
- Assignment operators
- Arithmetic operators
- Comparison operators
- Logical operators
- Bitwise operators
- Membership operators
- Identity operators
1.8.1 Assignment Operators:
Assignment operators are used for assigning the values generated after evaluating the right hand operand to the left hand operand. It always works from right to left. There are two types of assignment operators which are as follows.
- Simple Assignment Operator
- Compound Assignment Operator
- Simple Assignment Operator: Simple assignment is done with the equal sign (=) which simply assigns the value of its right operand to the variable on the left.
For example:
#Program for demonstration of receiving input from keyboard n1 = int(input(“Enter first number:”)) n2 = int(input(“Enter second number:”)) sum = n1 + n2 print (“Addition:”, sum) |
Output:
value of a = 3 value of b = 4 value of a = 4 value of b = 4 |
2. Compound Assignment Operator: They support shorthand notation for avoiding the repetition of the left-side variable on the right side. These compound assignment operators are also known as short-hand assignment operators.
For example: the statement
>>>x = x + 1
Can be written in compact form as shown below:
>>>x +=1 # It will add 1 to the variable x and later will assign the result to variable x.
Operator | Meaning |
+= | It adds right operand to the left operand and assign the result to left operand. |
-= | Its subtracts right operand from the left operand and assign the result to left operand. |
*= | It multiplies right opened with the left operand and assign the result to left operand. |
/= | It divides left operand with the right operand and assign the result to left operand. |
%= | It takes modulus using two operands and assign the result to left operand. |
//= | Performs power (exponential) calculation on operands and assign value to the left operand. |
**= | It performs floor division on operands and assign value to the left operand. |
1.8.2 Arithmetic operators:
Arithmetical operators are used to perform arithmetical operations like addition, subtraction, multiplication, division, etc.
Operator | Name | Meaning |
+ | Addition Operator | Add two operands |
– | Subtraction Operator | Subtract right operand from the left |
* | Multiplication Operator | Multiply two operands |
/ | The division Operator | Divide left operand by the right one and returns result into float |
% | Modulus Operator | Returns remainder of the division of left operand by the right. |
// | Floor Division Operator | Divide left operand by the right one and returns results into whole number adjusted to the left in the number line. |
** | Exponent Operator | Returns value of left operand raised to the power of right. |
1.8.3 Relational or Comparison operators:
Comparison operators are used to compare values on left hand side and right hand side and find relation between them.
Operator | Name | Meaning |
< | Less than operator | Returns True if left operand is less than the right. |
> | Greater than operator | Returns True if left operand is greater than the right. |
== | Equal to operator | Returns True if both operands are equal. |
!= | Not equal to operator | Returns True if both operands are not equal. |
>= | Greater than or equal to operator | Returns True if left operand is greater than or equal to right operand. |
<= | Less than or equal to operator | Returns True if left operand is Less than or equal to right operand. |
1.8.4 Logical operators:
Logical operators are used to combine two or more conditions and perform the logical operations using logical AND, logical OR and logical NOT.
Operator | Name | Meaning |
And | Logical AND operator | Returns True if both the operands returns true. |
Or | Logical OR operator | Returns True if either of the operand returns true. |
Not | Logical NOT operator | Returns True if operand is false and return False if operand is True. |
1.8.5 Bitwise Operators:
Bitwise operator works on bits and perform bit by bit operation. Assume if a=60; and b=13; Now in the binary format their values will be 0011 1100 and 0000 1101 respectively.
Operator | Name | Meaning |
& | Binary AND | Operators copies a bit to the result if it exists in both operands. |
| | Binary OR | It copies a bit if it exists in either operand. |
^ | Binary XOR | It copies a bit if it is set in one operand but not both. |
~ | Binary Ones Complement | It is unary and has the effect of flipping bits. |
<< | Binary Left Shift | The left operand value is moved left by the number of bits specified by the right operand. |
>> | Binary Right Shift | The left operand value is moved right by the number of bits specified by the right operand. |
1.8.6 Membership Operators:
Python’s membership operators test for membership in a sequence, such as strings, list, or tuples.
Operator | Meaning | Example |
in | Evaluate to true if it finds a variable in the specified sequence and false otherwise. | x in y, here in results in a 1 if x is member of sequence y. |
not in | Evaluates to true if it does not finds a variable in the specified sequence and false otherwise. | x not in y, here not in results in a 1 if x is not a member of sequence y. |
1.8.7 Identity Operators:
Identity operators compare the memory locations of two objects.
Operator | Meaning | Example |
is | Evaluate to True if the the variables on other side of the operator point to the same object and False otherwise. | X is y, here in results in I if id(x) equal id(y). |
is not | Evaluates to False if the variable on either side of the operator point to the same object and True otherwise. | x is not y, here is not results in I if id(x) is not equal to id(y). |
1.8.8 Python Operator Precedence and Associativity:
As we all know, an expression is a combination of operands, operators and constants (values). Now, consider the simple expression, print (17 + 10), Python interpreter evaluates it and then return 27 as output. Since there is just one operation to be performed, its output is straightforward. But, such calculations can get complex when 2 or more operators are involved in an expression. Let’s consider another expressions.
print (9 + 2 – 6 * 4)
How does it calculate?
Multiplication – Addition – Subtraction
6 * 4 = 24 —- 9 + 2 = 11 – 24 = – 13 output
Python Operator Precedence (Highest to Lowest)
Below is the table containing the precedence of all operators in Python. The operators are listed down in the decreasing order of precedence.
In a previous example, addition was performed before subtraction, but the precedence of plus and minus is the same. Then how did interpreter determine that addition has higher precedence than subtraction? That’s where the associativity of operators comes into the picture.
Operator | Name |
( ) | Parenthesis |
* * | Exponent |
~ | Bitwise NOT |
*, /, %, // | Multiplication, Division, Modulus, Floor Division |
+, – | Addition, Subtraction |
>>, << | Bitwise right and left shift |
& | Bitwise AND |
^ | BItwise XOR |
| | Bitwise OR |
==, !=, >, <, >=, <= | Comparison |
=, +=, -=, /=, %=, **=, //= | Assignment |
is, is not | Identity |
in, not in | Membership |
and, or, not | Logical |
Python Operator Associativity:
Associativity is the order in which an expression with multiple operators of the same precedence is evaluated. Associativity can be either from left to right or right to left. Almost all the operators have left-to-right associativity, except the few. For example, consider an expression having operators with the same precedence, print (a*b/c)
Here the multiplication * and division / operators have left to right associativity. This means a and bare multiplied first and the result thus obtained is then divided by b. This is simply because when we read the expression from left to right, multiplication comes first and hence it gets evaluated first. Below is the table containing the associativity of all operators in Python.
Operator | Name | Associativity |
( ) | Parenthesis | Left to right |
* * | Exponent | Right to left |
~ | Bitwise NOT | Left to right |
*, /, %, // | Multiplication, Division, Modulus, Floor Division | Left to right |
+, – | Addition, Subtraction | Left to right |
>>, << | Bitwise right and left shift | Left to right |
& | Bitwise AND | Left to right |
^ | BItwise XOR | Left to right |
| | Bitwise OR | Left to right |
==, !=, >, <, >=, <= | Comparison | Left to right |
=, +=, -=, /=, %=, **=, //= | Assignment | Right to left |
is, is not | Identity | Left to right |
in, not in | Membership | Left to right |
and, or, not | Logical | Left to right |
In the above given table, only exponent and assignment operators have the right to left associativity. All the other operators follow left to right associativity.
Frequently Asked Questions (FAQs)
- What is a docstring?
- What is the purpose of each operator in Python?
- Define the term keyboard and identifier.
- Write down the types of comment available in Python.
- Differentiate between expression and statement.
- How can we get the input from the user in Python program? Write down the different kinds of operators available in Python with example.
- Write to program to calculate the total and percentage of marks, where marks of three subjects will be entered through the keyboard.
- Write a program to calculate inverse of entered number.
- Write a program to calculate simple interest where principal amount, rate of interest will be entered through keyboard.
- Write a program to calculate the area of circle with radius of circle will be entered through the key.
Fundamentals of Python Programming
Read More: https://digitalcomputereducation.com/introduction-to-python-programming/
Know More: https://youtu.be/zrtgAQdrbr0
2 thoughts on “Fundamentals of Python Programming”